Web development with python and flask part 1
- winstonmhango23
- Jan 27, 2021
- 5 min read
In this part of our series, you will learn how to create project folders, virtual environments, install, create and run your very first Flask web application.

CONTENT LAY OUT
Creating a project directory and virtual environment
Installing flask
Creating a flask app
Running the flask app
Conclusion
1. CREATING A PROJECT DIRECTORY AND VIRTUAL ENVIRONMENT
First of all, we need to create our new project directory. We're going to a new directory called flask_app in our home directory. I strongly advise that you use the same names for your project so it's easier to follow along. Open Git bash(You have to search and install Git bash if you don't have it installed) in your documents folder and type in commands below:
mkdir flask_app
cd flask_app
touch app.py
virtualenv venv
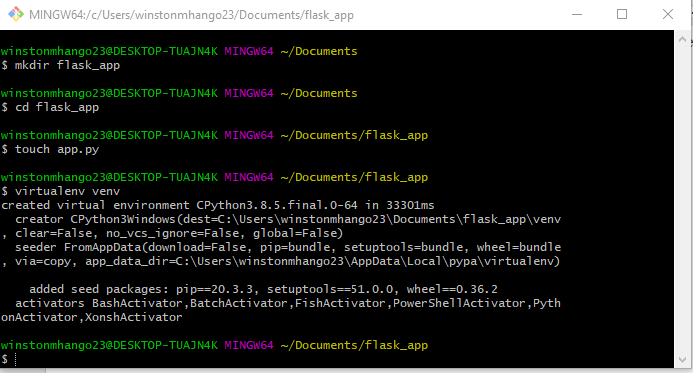
This will create the following files and folders:
1. mkdir flask_app : will create a directory named flask_app.
2. cd flask_app: changes you into the created directory(flask_app)
3. touch app.py : creates a file named app.py inside the current directory(flask_app)
4. virtualenv venv: creates a virtual environment named venv in the current folder.

Before we can install the required python flask module; we need to activate our virtual environment(venv) so the libraries get installed inside the venv directory.
By the way, a virtual environment helps us create separate python installation packages. The reason is to make sure we don’t pollute the python standard libraries and modules with third party modules and libraries that may have different version which may result into compatibility issues. To activate the virtual environment just type in the command shown below.
venv\scripts\activate
(venv) C:\Users\winstonmhango23\Documents\flask_app>
Once active, the venv folder is wrapped inside parentheses at the beginning of our folder structure in the console as shown in the second line on the script above(this is how it shows in my system...so names and structure may and will be named differently). Let’s check what is inside our virtual environment before we can install the flask package by using pip list comand
(venv) C:\Users\winstonmhango23\Documents\flask_app>pip list
package version
_________________________________
pip 20.3.3
setuptools 51.0.0
wheels 0.36.2
(venv) C:\Users\winstonmhango23\Documents\flask_app>
As you can see, there isn’t much. I will not go into discussing about the packages listed above. That is a topic we will talk about in our Python series right on this blog.
2. CREATING A FLASK APPLICATION
Let’s install our flask package now. You install flask just as you would any other Python package (pip install <package name>). Now go ahead in your console and run the command below:
(venv) C:\Users\winstonmhango23\Documents\flask_app>pip install flask
with that, we have our flask module installed in the venv (virtual environment) folder of our project. Running pip list again in the console, we should see flask, itsdangerous, jinja2,werkzeug ,MarkupSafe, and other packages that get installed along with it among the packages currently available in our environment as seen below.
(venv) C:\Users\winstonmhango23\Documents\flask_app>pip list
package version
_________________________________
pip 20.3.3
setuptools 51.0.0
wheels 0.36.2
click 7.1.2
Flask 1.1.2
itsdangerous 1.1.0
Jinja2 2.11.2
MarkUpsafe 1.1.1
Werkzeug 1.0.1
As from the start,the flask framework is built on top of the werkzeug tool and Jinja2 templating engine.It should therefore not be surprising to see them installed together with flask.As for other packages,we will into them as we progress in the series.
Now, we have everything we need to start creating our simple first flask application. Let’s get down with it.
3. CREATING A FLASK APPLICATION
Remember the app.py file we created using touch app.py command during the creation of our project folder and the virtual environment? That is the file we will be putting our flask scripts. Open it is your editor(sublime,visual studio code,etc) and start by importing the flask library in it like in the line below.
fromflaskimport Flask
If you are a python coder or at least have done OOP in any other language, the syntax here isn’t that strange. We are importing a class named Flask from the flask module. The next thing we need is to create the instance of the Flask class. We're going to pass __name__ to Flask and assign it to the variable app.
from flask import Flask
app = Flask(__name__)
It is very important that you understand that Flask(with capital “F”), is the most import part. It creates an object which refers to the entirety of the app itself. When we create an object app = Flask(__name__), we are creating a variable(which is an instance) that represents our application. When we configure the variable app, we are actually configuring the way our entire application will operate. The Flask() method can accept a number of attributes, in form of settings. For example, we can set the following values:
app = Flask( __name__,
instance_relative_config=False,
template folder="templates",
static folder="static" )
This sets a few setting specifics for our instance, namely the location of our config file, the folder where we will store our HTML template files, and the folder where we will store the front end assets like CSS, images, and JavaScript files.
Next up, we need to create a route together with its view function (route and view are used interchangeably many times)
from flask import Flask
app = Flask(__name__)
@app.route("/")
def index():
return"Hello world!"
What are these three lines doing?
@app.route("/")
The primary function of our app is called index(), which is importantly wrapped by Flask's most important decorator .route(). If you are not familiar with decorators in Python, a decorator is a function for us to wrap other functions with.
Flask comes with a route decorator which allows us to serve up functions based on which page of the app the user is loading. By setting @app.route("/"), we are specifying that the function index() should be executed whenever someone visits the root directory(www.yoursite.com) of our application.
In our case, the index() function returns a simple “Hello World” string. But honestly, there is where lies the power and real magic of flask. You can virtually return any values here. You can return complex calculations, HTML templates, data from databases, and much more complex python calculations. And that is what we will be doing in the coming episodes.
To run our application, we need two more lines of code:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def index():
return"Hello world!"
if __name__ == "__main__":
app.run()
Hey, what have we added here!?
if __name__ == "__main__":
app.run()
Take it easy, don’t worry too much about the whole syntax in the two lines here, we will get into all that as we progress in our series. For now, just know that __name__ is a special variable used by the Python interpreter to understand if a file is the main program.
Just as we passed __name__ into the Flask() class, the special variable __name__ is equal to __main__
4. RUNNING THE FLASK APPLICATION
Time to see the app in action! We can run our Flask app in a couple of ways. In your terminal, make sure you're in the same directory as app.py and run the following:
(venv) C:\Users\winstonmhango23\Documents\flask_app>python app.py
You should see the following in your terminal.

Our app is running. Open up a new web browser tab and type the following web address: http://127.0.0.1:5000/
You should see the following screen with a “Hello world” string in it.

Head back over to your terminal and stop the app by hitting Ctrl + c.
5. CONCLUTION
As we have observed, flask is just a module that at its core has a class named Flask. The class defines and has methods that create a server that handles requests from different view function through their decorator route wrappers. The view function returns templates and related python code to display in the route specified in the route decorator.
The best way to install flask and its associated packages and modules is through a virtual environment.
As stated,this is the beginning of a lengthy series of web development with python and flask. So you may want to Join a tech forum on this site to keep updated and be involved in the discussions about topics on this blog.
Comments